Angular Host Property: Hidden Feature Every Developer Should Know 🤫🤫
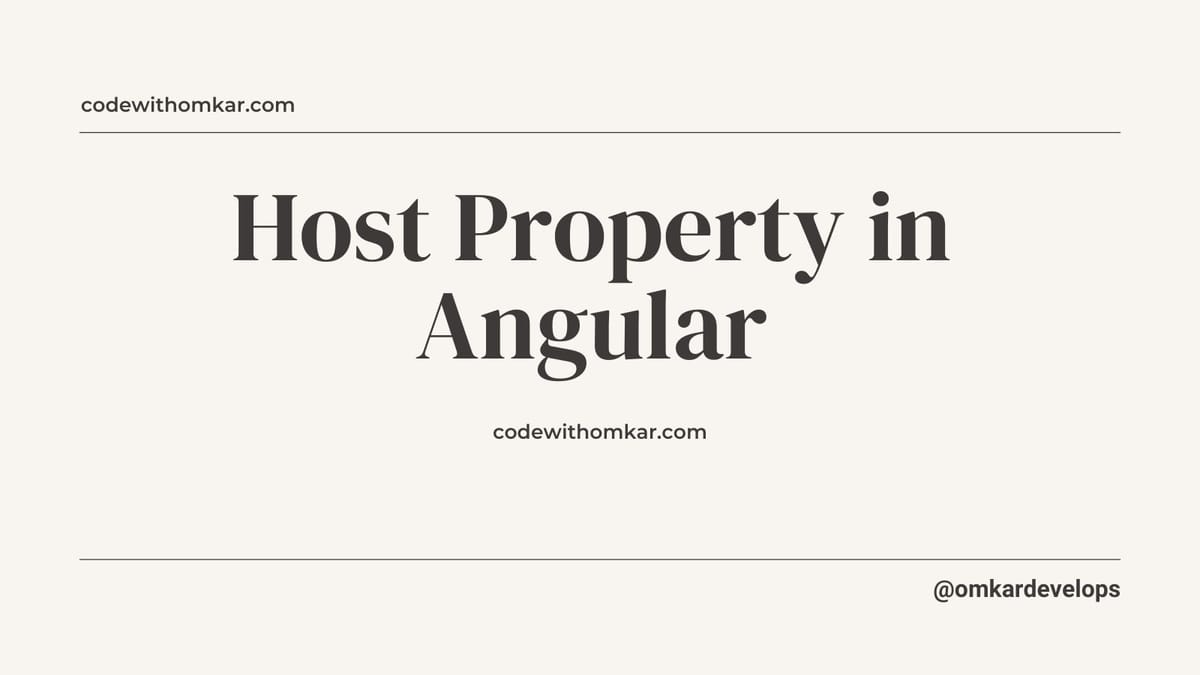
What is a host element?
Angular creates an instance of a component as soon as it sees a your component selector in DOM . The matching DOM element eg. app-card
in this case is your host element .

The template content of the component is then rendered inside the host element

Host Property
Using host property you can now bind properties , attributes , or events to your host element from the @Component
decorator itself
Let's look at an example :
@Component({
selector: 'app-custom-button',
standalone:true,
template: `
<ng-content></ng-content>
`,
styles: [`
:host {
display: inline-block;
padding: 8px 16px;
border-radius: 4px;
cursor: pointer;
transition: all 0.3s ease;
}
:host(.primary) {
background-color: #007bff;
color: white;
border: none;
}
:host(.disabled) {
background-color: #cccccc;
cursor: not-allowed;
opacity: 0.7;
}
:host(:hover):not(.disabled) {
transform: translateY(-2px);
box-shadow: 0 2px 4px rgba(0,0,0,0.2);
}
`],
host: {
'[class.primary]': 'variant === "primary"',
'[class.disabled]': 'disabled',
'[attr.disabled]': 'disabled ? "" : null',
'(click)': 'onClick($event)',
'role': 'button',
'[attr.aria-disabled]': 'disabled'
}
})
export class CustomButtonComponent {
@Input() variant: 'primary' | 'secondary' = 'primary';
@Input() disabled = false;
@Output() buttonClick = new EventEmitter<Event>();
onClick(event: Event) {
if (this.disabled) {
event.preventDefault();
event.stopPropagation();
return;
}
this.buttonClick.emit(event);
}
}
In the above example you can see I have added some attributes and events ([class.primary]
,(click)
) to my host element , by defining it in host property .
Angular recommends to use host
property instead of @HostBinding
and @HostListener
. These decorators exist exclusively for backwards compatibility.
Benefits:
- Cleaner component code
- Reduced template complexity and helps to give a clean template syntax
- No need for additional wrapper elements
- Better encapsulation of host element behaviour