Native Observables in Javascript
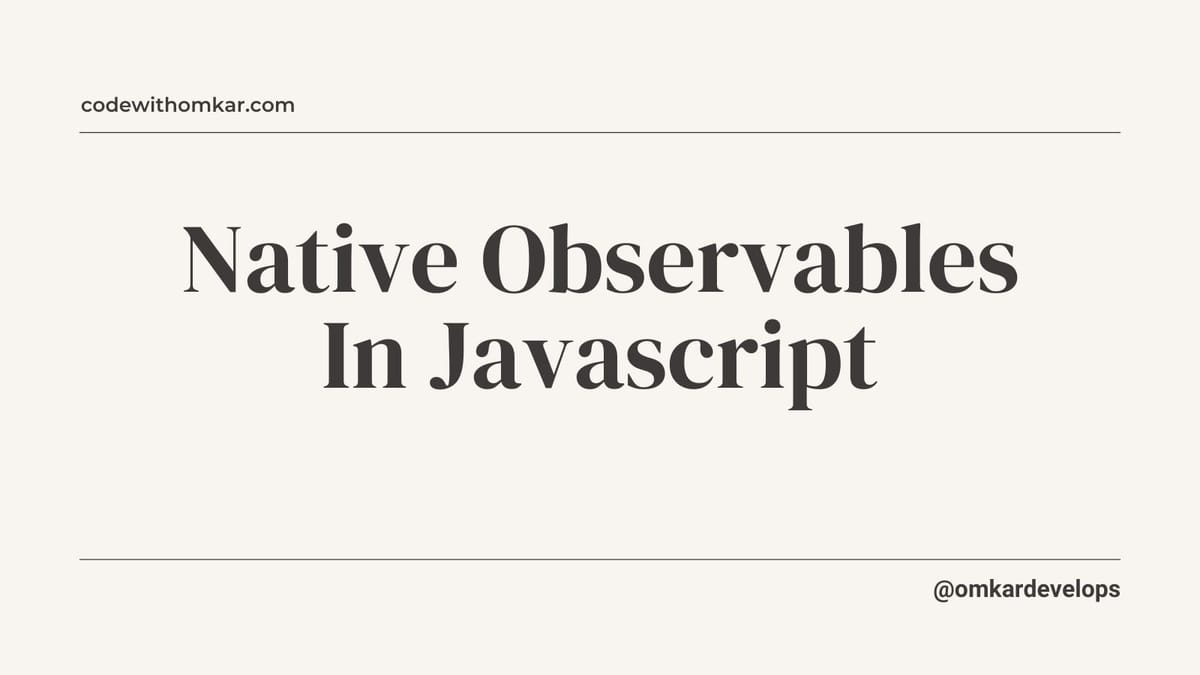
What are Observables ?
Imagine subscribing to my newsletter: you sign up once, and then you receive updates whenever they’re available— mostly on weekly basis . You don’t have to keep checking for new content; it’s delivered to you in your inbox . In programming terms, this is exactly what Observables do. They allow you to subscribe to a stream of data that arrives over time, such as user inputs, API responses, or real-time events. Observables are a programming design pattern for handling asynchronous data streams.
Native Observables in Chrome 135
Until recently, developers relied on libraries like RxJS to use Observables in JavaScript. But starting with Chrome 135, Observables are now a native feature of JavaScript. This means you can use them without any external dependencies, making your code lighter and more efficient. Native support also ensures better integrations and opening upon possibility on how we build applications .
Key Features of Native Observables
Native observables comes with some powerful features . Lets dive in and see them one by one :
Simple Subscription Model
You can subscribe to an Observable to receive its values, errors, or completion signals. It’s like tuning into a radio station—you get updates as they happen, and you can tune out when you’re done.
Its similar to the ones we use with help of RxJS
Multicasting by default
In world of observables there is multicasting and unicasting . Multicasting means that multiple subscribers can receive the same data from a single source. whereas unicasting means each subscriber to an observable gets its own data stream and the source it executed separately for each subscriber .
For native observables it multicasting by default , whereas in RxJS observables are unicast .
Built in Cancellation
Ever subscribed to a radio station and then decided you didn’t want to listen it to anymore?
Native Observables make it easy to cancel subscriptions using AbortController
. It's similar to that we have for unsubscribe()
in RxJS . This is helpful for cleaning up resources and avoiding memory leaks especially in big web applications .
Promise Integration
Native Observables play nicely with Promises, which is great news if you’re already comfortable with async/await
. Methods like first() last()
return Promises, making it seamless to integrate Observables into your existing code.
Native Observables vs RxJS
If you’ve used RxJS, you might be wondering how native Observables stack up. Here’s a quick comparison to help you decide when to use each:
Feature | Native Observables | RxJS |
---|---|---|
Multicasting | Automatic, like a radio broadcast | Manual setup with operators like share() |
Operators | Minimal tools, custom code for transformations | Rich library (map , filter , switchMap ) |
Browser Integration | Built for browser APIs (DOM, fetch , WebSockets) |
General-purpose, not browser-optimized |
Use Cases | Simple, browser-focused tasks (clicks, streaming) | Reactive UIs, complex logic (debouncing, APIs) |
Practical Example of Native Observables
Let's see how we can react to single button click
const button = document.getElementById("myButton")
const controller = new AbortController();
const clickObservable = new Observable(observer => {
const handler = () => {
observer.next('Hey! Someone clicked me ');
observer.complete(); // Stop after first click
};
button.addEventListener('click', handler, { signal: controller.signal });
return () => button.removeEventListener('click', handler);
});
clickObservable.subscribe({
next: message => console.log(message),
error: err => console.error('Error:', err),
complete: () => console.log('Stopped listening for clicks')
});
// Optional: Cancel manually after 10 seconds if no click occurs
setTimeout(() => controller.abort(), 10000);
The Observable
listens for a click
event, emits a message, and stops. The AbortController
ensures cleanup if you cancel early—like unsubscribing from a radio station . If multiple subscribers were added, they’d all receive the same click event, thanks to multicasting.
Possible Implications For Angular
If you’re an Angular developer, you’re probably familiar with RxJS—it’s deeply integrated into the framework for handling reactivity, from the AsyncPipe
to the HttpClient
. So, what do native Observables mean for Angular?
A Simpler Option?
Native Observables could simplify some tasks in Angular, especially when dealing with browser events or Web APIs. For example, you might use native Observables for straightforward event handling, reducing the need for RxJS in certain scenarios.
RxJS Stays Relevant
However, for most Angular developers, RxJS will remain essential. Its powerful operators and seamless integration with Angular’s core features mean that native Observables are more likely to complement RxJS rather than replace it. Think of native Observables as a new tool in your toolkit, not a replacement for the whole toolbox.
Conclusion and Way Forward
Native Observables are an exciting addition to JavaScript, offering a simple, more integrated way to handle asynchronous data streams. With features like default multicasting, built-in cancellation, and Promise integration, they make async programming more accessible and efficient.
Why It’s Exciting
- Simplicity: Fewer concepts to learn, especially for developers who are used to RxJs .
- Native Support: No external libraries needed, leading to lighter, faster applications.
- Better Browser Integration: Deep ties with Web APIs make them a natural fit for modern web development.
Get Started
If you are eager to try out the new native Observables , you can experiment it with Chrome 135 or later .
Way Ahead
The future of native Observables is still unfolding, and the TC39 proposal is worth keeping an eye on for updates. You can track its progress here.
Stay in the loop with the latest in JavaScript, TypeScript, and Angular! Subscribe to my newsletter and never miss a blog post, tip, or free resource to boost your frontend skills.